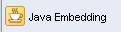
/* Start of Code snippet */
/*Write your java code below e.g.
System.out.println("Hello, World");
*/
try{
String status = "Bronze";
/* accessing a process variable of type string */
String country = (String)getVariableData("v_country");
/* accessing a variable within the complex type - customer */
Element country1 = (Element)getVariableData("inputVariable", "payload","/ns1:customer/ns1:Country");
String c1 = country1.getTextContent();
/* writes to the audit trail - will then be visible in BPEL console at runtime*/
addAuditTrailEntry("country is: " + country);
addAuditTrailEntry("country1 is: " + c1);
if (country.equalsIgnoreCase("Ireland")){
status = "Gold";
}
/* setting the output variable within the complex type - customer */
setVariableData("outputVariable", "payload",
"/ns1:customer/ns1:Status", status);
addAuditTrailEntry("status is: " + status);
}
/* End of Code snippet */
catch (Exception e) {
addAuditTrailEntry(e);
}
Add the following line before the bpelx:exec tag to import the Element class

Calling an external Java class in a Java activity
Jar up the Java class you want to use and copy the jarfile to the BPEL
system\services\lib directory.
In this example, my class is called CustomerStatus
/* Start of Code snippet */
try{
String status = "Bronze";
String country = (String)getVariableData("v_country");
Element country1 = (Element)getVariableData("inputVariable", "payload","/ns1:customer/ns1:Country");
String c1 = country1.getTextContent();
addAuditTrailEntry("country is: " + country);
addAuditTrailEntry("country1 is: " + c1);
CustomerStatus cs = new CustomerStatus();
status = cs.getStatus(country);
setVariableData("outputVariable", "payload",
"/ns1:customer/ns1:Status", status);
addAuditTrailEntry("status is: " + status);
}
catch (Exception e) {
addAuditTrailEntry(e);
}
/* End of Code snippet */
add the following entries before the bpelx:exec tag
