In this lab I will leverage the ADF Hierarchy Viewer to display data on Countries and Regions in an innovative way.
Pre-Requisites
- 2 DB Tables --> Country, Region
CREATE TABLE COUNTRY
(
COUNTRY VARCHAR2(20 BYTE) NOT NULL,
POPULATION NUMBER(10, 0) NOT NULL,
CAPITAL VARCHAR2(100 BYTE) NOT NULL,
GNP VARCHAR2(20 BYTE) NOT NULL,
BURGER_INDEX NUMBER(3, 2) NOT NULL,
IMAGE BFILE,
COMMENTS VARCHAR2(100 BYTE)
, CONSTRAINT COUNTRY_PK PRIMARY KEY
(
COUNTRY
)
ENABLE
)
TABLESPACE "USERS"
LOGGING
PCTFREE 10
INITRANS 1
MAXTRANS 255
STORAGE
(
INITIAL 64K
MINEXTENTS 1
MAXEXTENTS 2147483645
BUFFER_POOL DEFAULT
)
;
CREATE TABLE REGION
(
REGION VARCHAR2(100 BYTE) NOT NULL,
COUNTRY VARCHAR2(20 BYTE) NOT NULL,
CAPITAL VARCHAR2(100 BYTE) NOT NULL,
IMAGE BFILE,
MOST_SCENIC_SITE VARCHAR2(100 BYTE),
COMMENTS VARCHAR2(255 BYTE)
, CONSTRAINT REGION_PK PRIMARY KEY
(
REGION
)
ENABLE
)
TABLESPACE "USERS"
LOGGING
PCTFREE 10
INITRANS 1
MAXTRANS 255
STORAGE
(
INITIAL 64K
MINEXTENTS 1
MAXEXTENTS 2147483645
BUFFER_POOL DEFAULT
)
;
ALTER TABLE REGION
ADD CONSTRAINT REGION_COUNTRY_FK FOREIGN KEY
(
COUNTRY
)
REFERENCES COUNTRY
(
COUNTRY
)
ON DELETE CASCADE ENABLE
;
Add Some Test Data, I'm using jpgs for Ireland and its 4 regions
– In SQLPLUS -->
Create or replace directory imagedir
AS 'D:\fmw11g\demos\ADF-General\images';
insert into country values (’Ireland’, 4000000, ’Dublin’, 200, 4.5,
bfilename ('IMAGEDIR', 'Ireland.JPG'), ’ Great Place to Live! ’);
insert into region values (’Leinster’, ’Ireland’, ’Dublin’,
bfilename ('IMAGEDIR', 'Leinster.JPG'), ’ Hill of Howth ’,
’ Well worth a visit! ’);
insert into region values (’Ulster’, ’Ireland’, ’Belfast’,
bfilename ('IMAGEDIR', 'Ulster.JPG'), ’ Giants Causeway’,
’ Well worth a visit! ’);
insert into region values (’Munster’, ’Ireland’, ’Cork’,
bfilename ('IMAGEDIR', 'Munster.JPG'), ’ Ring of Kerry ’,
’ Well worth a visit! ’);
insert into region values (’Connaught’, ’Ireland’, ’Galway’,
bfilename ('IMAGEDIR', 'Connaught.JPG'), ’ Connemara’,
’ Well worth a visit! ’);
Check in JDev DB Browser
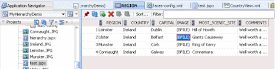
Create the ADF App
1. Create an ADF app of type – Fusion Web Application (ADF)
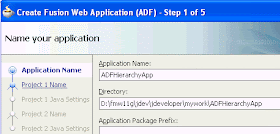
1.1. Accept defaults on the next screens.
2. Right-mouse click on the Model project
2.1. New...
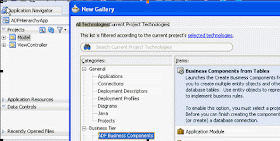
2.1.1. Create a new DB connection for scott
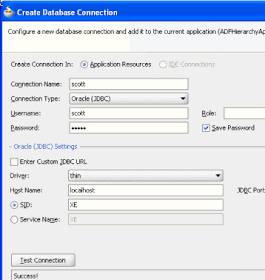
2.1.2. Select Entity Objects
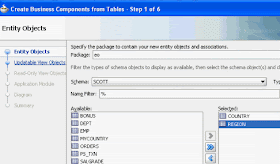
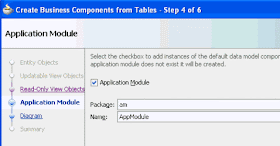
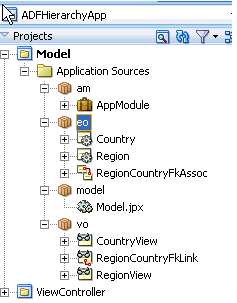
3 Add a Servlet to retrieve the BFile Images
3.1. Right-mouse click on the ViewController project and select New...
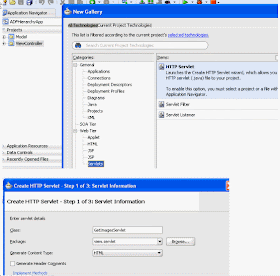
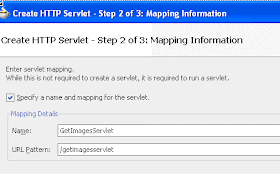
package view.servlet;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.servlet.*;
import javax.servlet.http.*;
import oracle.jbo.ApplicationModule;
import oracle.jbo.Row;
import oracle.jbo.ViewObject;
import oracle.jbo.client.Configuration;
import oracle.jbo.domain.BFileDomain;
import oracle.jbo.domain.BlobDomain;
public class GetImagesServlet extends HttpServlet { private static final String CONTENT_TYPE = "image/jpeg; charset=windows-1252";
public void init(ServletConfig config) throws ServletException {
super.init(config);
}
public void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
response.setContentType(CONTENT_TYPE);
/* here we will pass in the country / region as key for image retrieval */
String country = request.getParameter("Country");
String region = request.getParameter("Region");
String where = "";
OutputStream os = response.getOutputStream();
// get a handle to the Application Module
String amDef = "am.AppModule";
String config = "AppModuleLocal";
ApplicationModule am = Configuration.createRootApplicationModule(amDef, config);
ViewObject vo = am.findViewObject("CountryView1");
BFileDomain image = new BFileDomain();
// set where clause for country or region and get approp. View
if (country != null){
where = "country = " + "'"+ country +"'";
vo.setWhereClause(where);
vo.executeQuery();
}
if (region != null){
where = "region = " + "'"+ region +"'";
vo = am.findViewObject("RegionView1");
vo.setWhereClause(where);
vo.executeQuery();
}
if(vo.getEstimatedRowCount() != 0){
Row row = vo.first();
image = (BFileDomain)row.getAttribute("Image");
image.openFile();
InputStream is = image.getInputStream();
// copy bfile to output
byte[] buffer = new byte[10 * 1024];
int nread;
while ((nread = is.read(buffer)) != -1)
os.write(buffer, 0, nread);
os.close();
}
vo.setWhereClause(null);
Configuration.releaseRootApplicationModule(am, false);
}
}
4. Create a jsp to display the Country hierarchy
4.1 Double click on faces-config.xml
4.2 Drop a JSF page icon onto the designer --> Rename to hierarchy.jspx
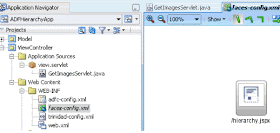
4.3 Double click on hierarchy.jspx to create the page
4.4. Open the Data Controls
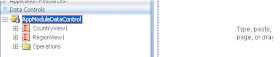
4.5 Drop CountryView1 as Hierarchy Viewer...
Radial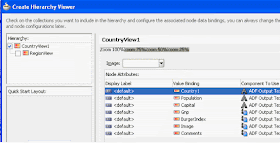
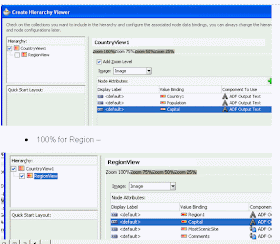
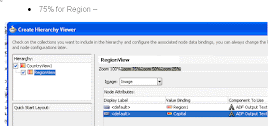
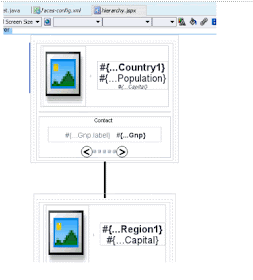
No comments:
Post a Comment